NeoPixel addressable LEDs
Light Emitting Diodes, or LEDs! Bright, colourful fun things that at first impression comes across as the equivalent of creating your very first drop shadow in Photoshop – but that just means you haven’t experimented with the nature of light itself!
NeoPixels are also known as WS2812B pixels. NeoPixel is the ‘brand’ given by Adafruit, a popular electronic prototyping store based in New York City, US. WS2812B is the actual part number of the addressable LED chips. They operate at 5VDC and will not do well if over/underpowered.
NeoPixels are addressable LED strips. They come in various form factors: individual chips, strips, rings and matrices. These can be ‘daisy-chained’ to achieve custom shapes, and physical patterns.
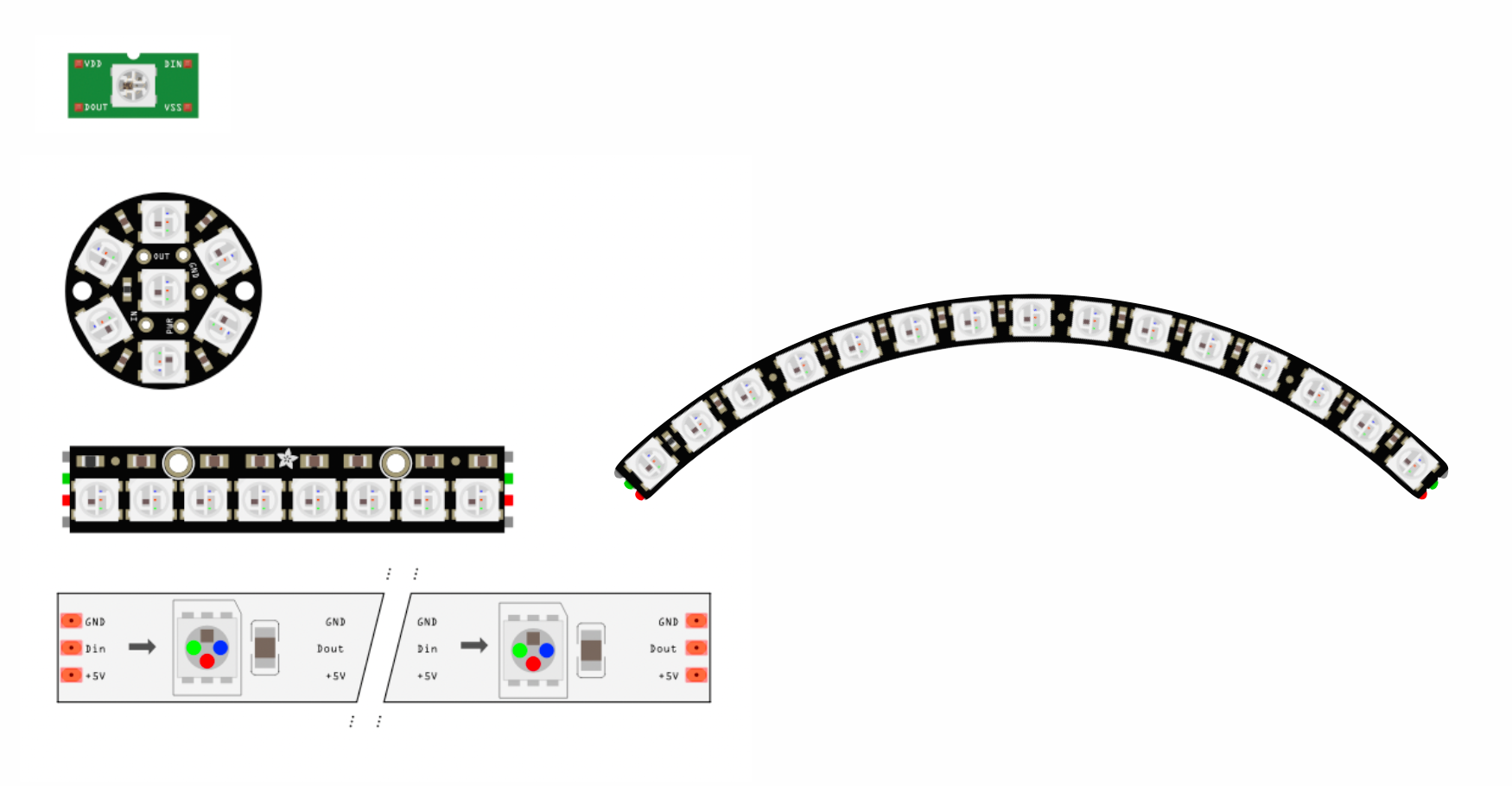
Different form factors of NeoPixels
The way we ‘address’ NeoPixels is through a numbered index. The first LED will be allocated an address position of 0
, and keep incrementing until the end of the strip is reached (effectively, an index of n-1
).

How we ‘address’ pixels on a NeoPixel strip
In the Arduino UNO code example below, we use the following command to assign an RGB colour value to an LED:
strip.setPixelColor(i, c);
Where
i
is the index position, andc
is the colour value to assign:
strip.setPixelColor(3, strip.Color(255, 0, 0));
In the above example, we will be writing a full red colour to pixel number 4 on the strip.
As you can see, NeoPixels are very different to the ‘garden variety’ LEDs that you might find in your kits, or that of long 12V RGB LED strips. A crucial differentiator between 12V ‘home improvement’ RGB strips and ‘addressable’ versions (the NeoPixels, WS2812B etc) is that you can change the colour output of a 12V RGB strip ALL AT ONCE, but not individually. That’s where the ‘addressable’ part of NeoPixels come in.
There’s not much point in repeating the excellent information that’s already written by Adafruit. For more information on NeoPixels, check the Neopixel Überguide here.
Other addressable LEDs
You might also have come across DotStar addressable LEDs, which use the APA102C chipset. A cheaper knockoff version of the APA102C is the SK9822. All of these different addressable LED technologies will require a a library that supports driving them. Once you get past the basics, you might be interested in the more versatile and full-featured FastLED library as an all-in-one solution for driving any of these addressable LED pixels.
In addition, you can also refer to the Low Frequency Oscillator (LFO) Coding Concept for a more advanced example of working with NeoPixels.
Power Considerations
A typical Neopixel will draw up to 60mA (0.06A). An 8-Neopixel stick will draw up to 480mA (0.48A) at full white brightness. Just like the 12V RGB LED strips, power consumption adds up, so make sure you choose the right 5V power supply. We’ll get by with the USB port’s 500mA in this recipe, but you definitely want to use either a wall-wart transformer, or one of those USB portable power banks for your phones if you want a more robust setup.
Wiring & Code
For a refresher on how to use the code in this recipe, click here.
For this recipe, we will use a potentiometer to adjust a parameter of the NeoPixels. To keep things simple, we will play with the red, green and blue channels of the entire NeoPixel strip. Check the code for more details.
-
Breadboard diagram
NeoPixels and potentiometer circuit
Download NeoPixels Fritzing file
Libraries Used
Adafruit_NeoPixel
You’ll need to install this from the Library Manager
Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73
#include <Adafruit_NeoPixel.h> // IMPORTANT: Set pixel COUNT, PIN and TYPE #define PIXEL_PIN 12 #define PIXEL_COUNT 8 #define PIXEL_TYPE WS2812B Adafruit_NeoPixel strip(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800); // code in this setup function runs just once, when Photon is powered up or reset void setup() { Serial.begin(115200); // Open a connection via the Serial port – useful for debugging pinMode(PIXEL_PIN, OUTPUT); // goes to Neopixel stick strip.begin(); strip.show(); // Initialize all pixels to 'off' delay(5000); // Common practice to allow board to 'settle' after startup } void loop() { updateLED(); } void updateLED() { // in this example we use a potentiometer on A0 to drive the Neopixels int sensorReading = analogRead(A0); int scaled = map(sensorReading, 0, 1023, 0, 255); // tweak these numbers accordingly! scaled = constrain(scaled, 0, 255); // make sure scaled ranged is kept within range // swap the 0 and scaled positions to test the (R,G,B) combinations. Here we are altering the Blue channel: colorAll(strip.Color(0, 0, scaled), 1); Serial.println(scaled); } // Set all pixels in the strip to a solid color, then wait (ms) void colorAll(uint32_t c, uint8_t wait) { uint16_t i; for(i=0; i<strip.numPixels(); i++) { strip.setPixelColor(i, c); } strip.show(); delay(wait); } /* Please note that the code provided here is licensed under the MIT license. The MIT License (MIT) Copyright © 2025 Chuan Khoo Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. */
-
COMING SOON…
More code samples for this component for other platforms will be added here over time.