Exertion / Mechanical
Distance
The VL53L1X IR laser Distance Rangefinder (whew!) is one of the more popular distance measurement sensors in recent times. It allows distance measurement in the range of 40-4000mm.
Utilising the Time-of-Flight (ToF) concept, it fires off a light point and measures the time taken for the reflection to return. The VL53L1X uses a low-power infra-red (IR) laser point which makes it invisible, and the form factor is much smaller compared to its predecessors such as the IR LED Rangefinder, or the even bulkier (and more error-prone) ultrasonic rangefinder. The reliability of the ToF laser rangefinder has surpassed these older technologies, and if your project requires narrow-beam distance sensing, this sensor is the way to go.
We are using Core Electronics’ version of the VL53L1X sensor for this recipe (follow the link to learn more details)
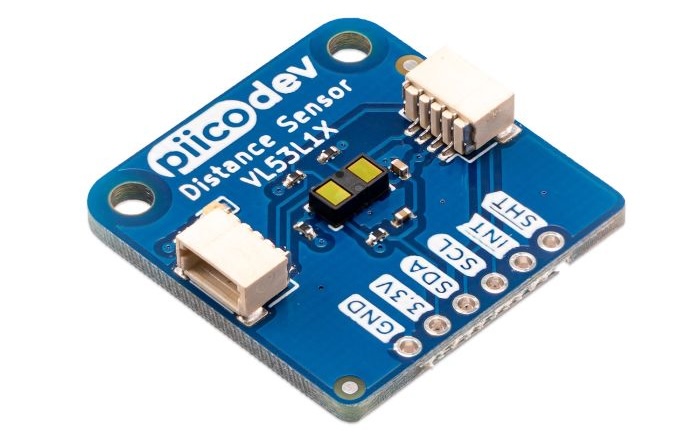
Piicodev Distance Laser Rangefinder VL53L1X.
I2C connection standards?
An increasing number and variety of sensors now use the I2C communication bus. Simply put, I2C sensors provide an easy way to connect it to a microcontroller.
The PiicoDev wiring is similar to a growing class of sensor interconnect standards such as Adafruit’s STEMMA, the identical pin order of Sparkfun’s QWIIC, or the slightly larger SeeedStudio’s Grove. Most of them are pin-compatible, but require specialty connector ends to fit. The healthy competition keeps innovation alive, but it can be slightly annoying finding the right cables to fit.
For PiicoDev sensors, you can connect the cable into either side of the sensor board. However the cable plug itself only goes into the socket one way, so exercise caution when connecting them.
Wiring & Code
Select a microcontroller platform in the tabs below to view the wiring and code meant for the platform:
-
Breadboard diagram
Distance sensor & LED circuit
Download Distance Fritzing file
Libraries
Install the following libraries via your Arduino IDE’s Library Manager, if you haven’t already:
- Adafruit VL53L1X
Code
For a refresher on how to use the code in this recipe, click here.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107
/* Distance Sensor (Time-of-Flight Laser) We are using the Core Electronics version of the VL53L1X, but the code library is referencing an Adafruit Library. It's a good point to know – that sensors can be assembled by various manufacturers, but so long as the sensor model number is the same, you are likely to get compatibility with code libraries written for the same sensor. This sensor returns accurate millimeter distance readings of objects directly in the path of the sensor. It fires off an invisible infra-red laser point and measures the time it takes for the reflected point to be detected. The angle of vision of this sensor is narrow; think of an invisible laser pointer. As it is a low-powered laser, it is not likely to cause any damage to the naked eye. Adjust the parameters below to set up a range of distances to dim the brightness of the LED. (Read the comments below for more details) */ #include "Adafruit_VL53L1X.h" #define IRQ_PIN 2 #define XSHUT_PIN 3 #define LED_PIN 11 // we are using the PWM-capable digital pin 11 to drive the brightness of an LED (on the UNO you can alternatively use 3, 5, 6, 9, 10, 11) ////// CUSTOMISE PARAMETERS HERE // Change these values to suit your sensing application needs. // Upload your sketch to the UNO after every edit, and observe the changes in sensitivity. #define TIMING_BUDGET 50 // Valid timing budgets: 15, 20, 33, 50, 100, 200 and 500ms. Quicker = less accurate #define DIST_MAX 1000 // set the maximum distance (in mm) you want to detect (4000mm, i.e. 4m, is max) #define DIST_MIN 40 // set the minimum distance (in mm) – minimum is 40mm #define LED_MINBRIGHT 0 // minimum brightness value of your LED (0-255) #define LED_MAXBRIGHT 255 // maximum brightness value of your LED (0-255) ////// END CUSTOMISATION OPTIONS Adafruit_VL53L1X vl53 = Adafruit_VL53L1X(XSHUT_PIN, IRQ_PIN); void setup() { Serial.begin(115200); while (!Serial) delay(10); Wire.begin(); if (!vl53.begin(0x29, &Wire)) { Serial.print("Error initialising VL sensor: "); Serial.println(vl53.vl_status); while (1) delay(10); } Serial.println("VL53L1X sensor OK!"); Serial.print("Sensor ID: 0x"); Serial.println(vl53.sensorID(), HEX); if (!vl53.startRanging()) { Serial.print("Couldn't start ranging: "); Serial.println(vl53.vl_status); while (1) delay(10); } Serial.println("Ranging started"); vl53.setTimingBudget(TIMING_BUDGET); Serial.print(F("Timing budget (ms): ")); Serial.println(vl53.getTimingBudget()); } void loop() { int16_t distance; if (vl53.dataReady()) { // new measurement for the taking! distance = vl53.distance(); if (distance == -1 || distance == 0) { // something went wrong! Most of the time it's when a subject has entered the laser's beam suddenly // and as a result the sensor requires a bit more time to readjust. // this has been commented out to keep the Serial printout simple // Serial.print("Couldn't get distance: "); // Serial.println(vl53.vl_status); return; } Serial.println(distance); // this is expressed in mm // data is read out, time for another reading! vl53.clearInterrupt(); } } /* Please note that the code provided here is licensed under the MIT license. The MIT License (MIT) Copyright © 2025 Chuan Khoo Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. */
-
COMING SOON…
More code samples for this component for other platforms will be added here over time.